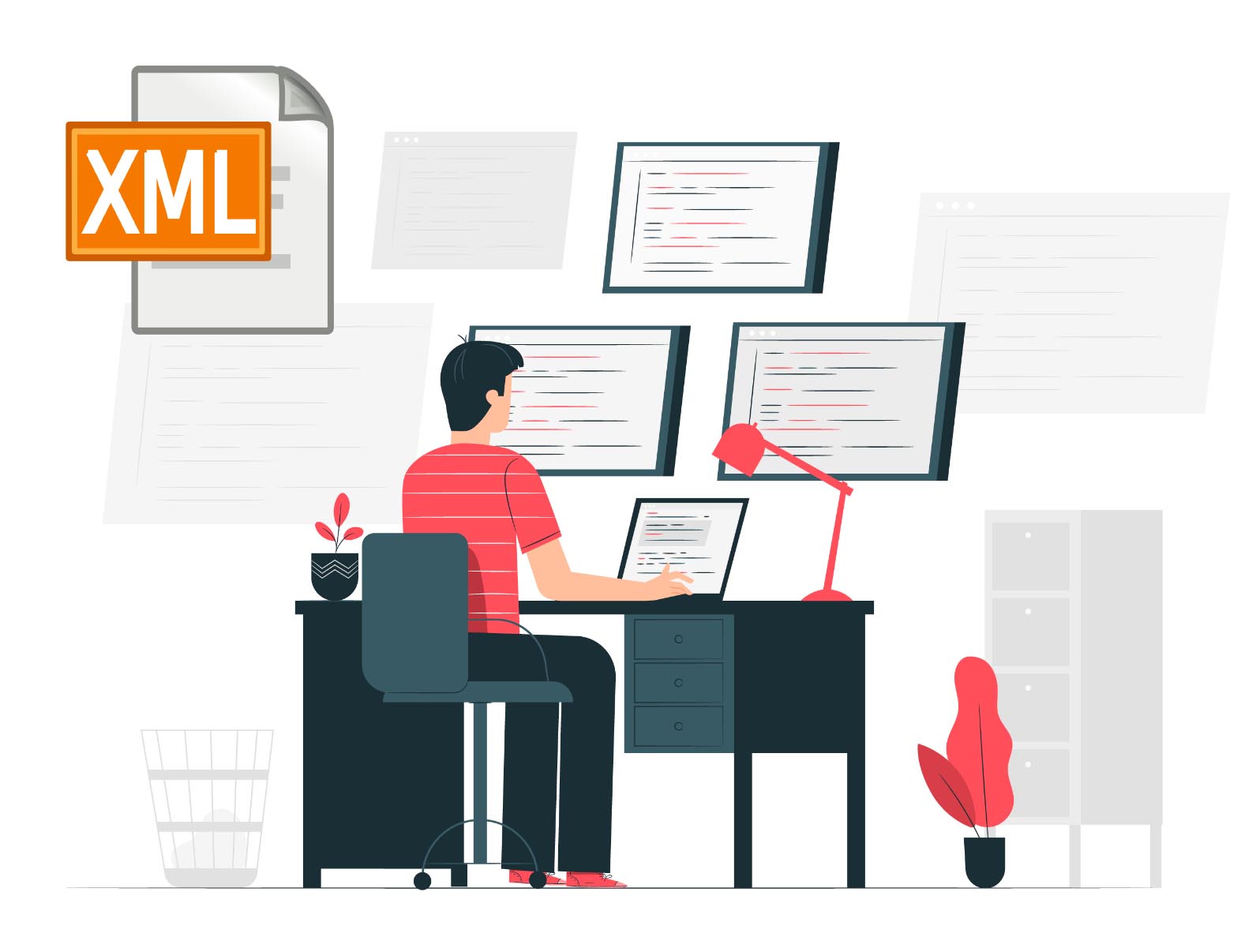
How to Parse a XML in Java
In this article you will learn how to Parse a XML in java. We are following below steps to Parse the XML.
- Create Document Builders to Retrieve XML Data
- Retrieve Root Element
- Retrieve Sub Elements
- Retrieve Child Elements
- Retrieve Attribute
Below is the XML file data which we are going to parse
<soup_menu>
<food index = "393">
<name>Soup 01</name>
<price>$1.23</price>
<description>Homemade Soup 01</description>
<calories>200</calories>
</food>
<food index = "394">
<name>Soup 02</name>
<price>$5.34</price>
<description>Homemade Soup 02</description>
<calories>300</calories>
</food>
<food index = "395">
<name>Soup 03</name>
<price>$5.33</price>
<description>Homemade Soup 03</description>
<calories>400</calories>
</food>
<food index = "396">
<name>Soup 04</name>
<price>$6.77</price>
<description>Homemade Soup 04</description>
<calories>500</calories>
</food>
<food index = "397">
<name>Soup 05</name>
<price>$3.12</price>
<description>Homemade Soup 05</description>
<calories>650</calories>
</food>
</soup_menu>
Import the related packages as below
import java.io.File;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
Add a Document Builder to Retrieve XML Data
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Parse the file
Document doc = dBuilder.parse(fXmlFile);
Retrieve the Root Element (soup_menu)
doc.getDocumentElement().getNodeName()
Retrieve the Sub Elements (food)
NodeList nList = doc.getElementsByTagName("food");
Retrieve the Attribute of Sub Element
System.out.println("Food Index # : " + eElement.getAttribute("index"));
Retrieve Child Elements
System.out.println("Name : " + eElement.getElementsByTagName("name").item(0).getTextContent());
System.out.println("Price : " + eElement.getElementsByTagName("price").item(0).getTextContent());
System.out.println("Description : " + eElement.getElementsByTagName("description").item(0).getTextContent());
System.out.println("Calories : " + eElement.getElementsByTagName("calories").item(0).getTextContent());
Full source code is as below
package xmlreader;
import java.io.File;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
*
* @author TechProgramme
*/
public class XMLReader {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
try {
File fXmlFile = new File("C:\\Users\\Sithara\\Documents\\NetBeansProjects\\menu.xml");
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(fXmlFile);
doc.getDocumentElement().normalize();
System.out.println("Root element :" + doc.getDocumentElement().getNodeName());
NodeList nList = doc.getElementsByTagName("food");
System.out.println("----------------------------");
for (int temp = 0; temp < nList.getLength(); temp++) {
Node nNode = nList.item(temp);
System.out.println("\nCurrent Element :" + nNode.getNodeName());
if (nNode.getNodeType() == Node.ELEMENT_NODE) {
Element eElement = (Element) nNode;
System.out.println("Food Index # : " + eElement.getAttribute("index"));
System.out.println("Name : " + eElement.getElementsByTagName("name").item(0).getTextContent());
System.out.println("Price : " + eElement.getElementsByTagName("price").item(0).getTextContent());
System.out.println("Description : " + eElement.getElementsByTagName("description").item(0).getTextContent());
System.out.println("Calories : " + eElement.getElementsByTagName("calories").item(0).getTextContent());
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Download the full project : https://github.com/TechProgramme/XMLReader