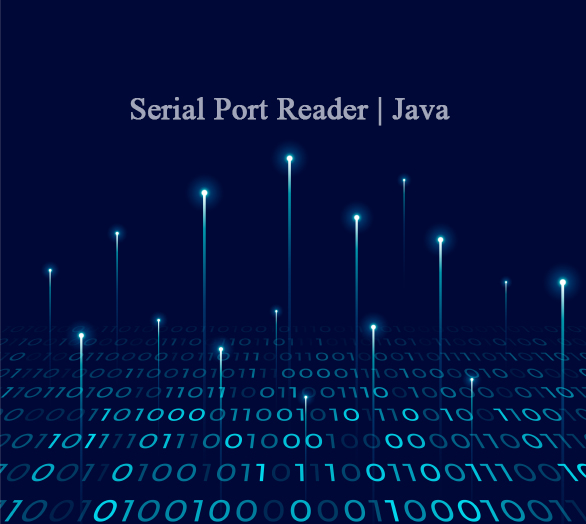
Read and Write to Serial Port Using JSSC Library in Java
JSSC (Java Simple Serial Connector) is a lightweight Java library which built to write and read data from Serial Ports from a Java Application. For this tutorial we have used the latest version 2.9.2 of the JSSC library which we can get to date.
This code line initializing the COM port
serialPort = new SerialPort("COM1");
This line is opening the COM Port connection
serialPort.openPort();
Below code is setting the parameters for serial access.
serialPort.setParams(SerialPort.BAUDRATE_9600,
SerialPort.DATABITS_8,
SerialPort.STOPBITS_1,
SerialPort.PARITY_NONE);
Below code managing the rate of data transmission via flow-control
serialPort.setFlowControlMode(SerialPort.FLOWCONTROL_RTSCTS_IN
| SerialPort.FLOWCONTROL_RTSCTS_OUT);
Once the application opened the port to receive data we can implement a listener for that. For this we are using an event reader as below
serialPort.addEventListener(new PortReader(), SerialPort.MASK_RXCHAR);
Same as reading data from serial port we can send data to the COM Port using below code lines
String s = "Hello World \n";
serialPort.writeString(s);
Below is the event listener for the COM Port and once a data revised to the Serial Port this event listener will trigger.
private class PortReader implements SerialPortEventListener {
@Override
public void serialEvent(SerialPortEvent event) {
if (event.isRXCHAR() && event.getEventValue() > 0) {
try {
String receivedData = serialPort.readString(event.getEventValue());
System.out.println("Recvied Data : " + receivedData);
} catch (SerialPortException ex) {
System.out.println("Error in receiving response from port: " + ex);
}
}
}
}
You can refer the full source code as below. You can use the same class to read/write to concurrent COM ports as well.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package serialreader;
import jssc.*;
/**
*
* @author Sithara
*/
public class SerialDataReceiver implements Runnable {
private SerialPort serialPort;
private void initCOMPort() {
serialPort = new SerialPort("COM1");
try {
serialPort.openPort();
serialPort.setParams(SerialPort.BAUDRATE_9600,
SerialPort.DATABITS_8,
SerialPort.STOPBITS_1,
SerialPort.PARITY_NONE);
serialPort.setFlowControlMode(SerialPort.FLOWCONTROL_RTSCTS_IN
| SerialPort.FLOWCONTROL_RTSCTS_OUT);
serialPort.addEventListener(new PortReader(), SerialPort.MASK_RXCHAR);
String s = "Hello World \n";
serialPort.writeString(s);
} catch (SerialPortException ex) {
System.out.println("Error in writing data to port: " + ex);
}
}
@Override
public void run() {
initCOMPort();
}
private class PortReader implements SerialPortEventListener {
@Override
public void serialEvent(SerialPortEvent event) {
if (event.isRXCHAR() && event.getEventValue() > 0) {
try {
String receivedData = serialPort.readString(event.getEventValue());
System.out.println("Recvied Data : " + receivedData);
} catch (SerialPortException ex) {
System.out.println("Error in receiving response from port: " + ex);
}
}
}
}
}