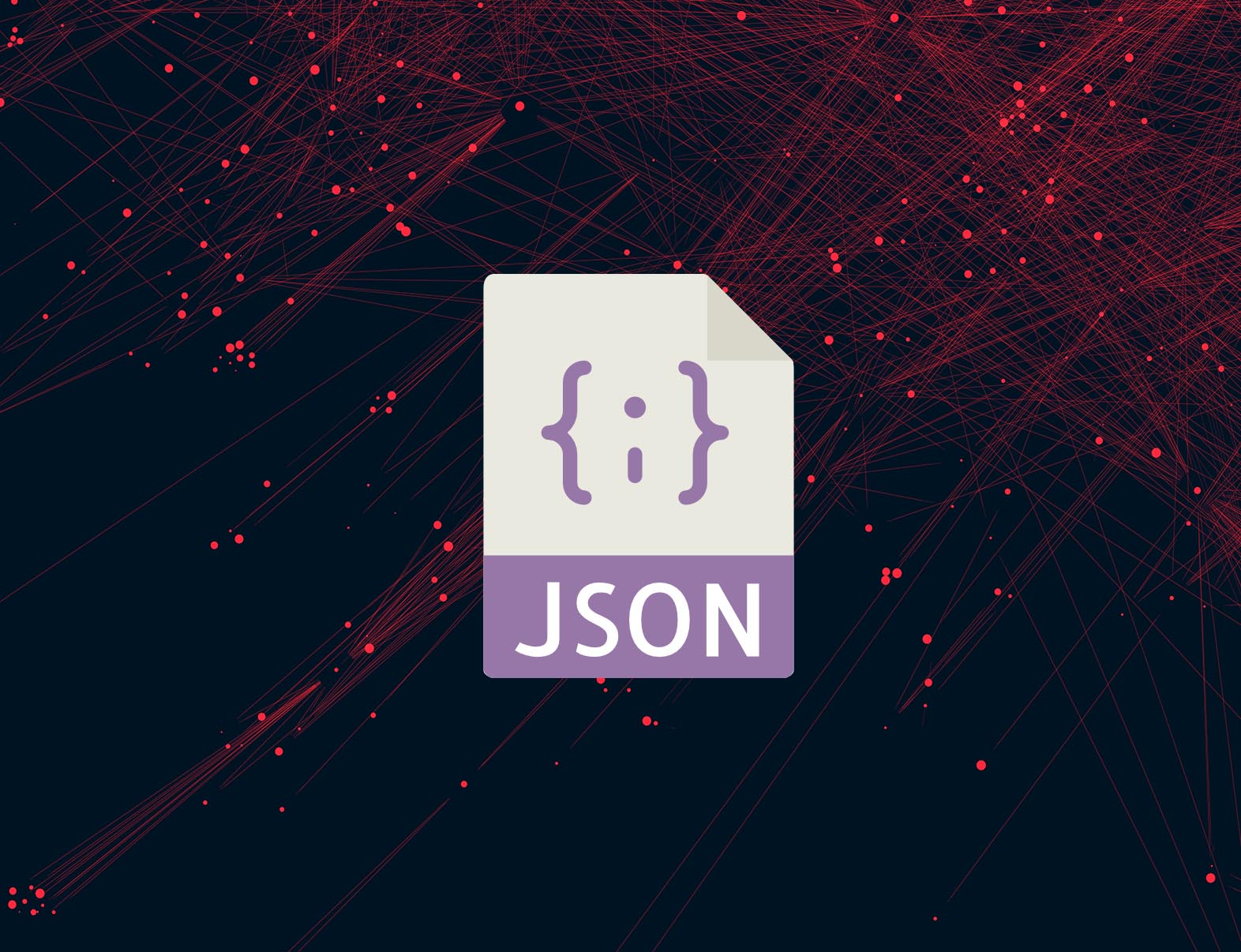
How to read a JSON file in java
Json is the mostly used data interchangeable format when comes to software developments. In this article you will learn how to read a JSON file in java. for this tutorial we are using the gson library. gson is an open source java library which allows to serialize and deserialize java objects to json and wise versa.
What you need :
Gson library (Download from here)
JDK 1.8 or later
Maven 3.2 +
Intelij or any IDE you prefer
Follow below steps to proceed with the programme
- Create a new Json file artist_json.json and add below Json content
{
"artist_id":"0001",
"artist_name":"Artist 01",
"artist_category":"Rock",
"artist_rating":"10",
"songs":[
{
"song_id":"1001",
"song_name":"Songs 01"
},
{
"song_id":"1002",
"song_name":"Songs 02"
},
{
"song_id":"1003",
"song_name":"Songs 03"
},
{
"song_id":"1004",
"song_name":"Songs 04"
}
]
}
2. Create a new Maven Project and add the Gson dependency to the pom.xml like below. get the latest library from here
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version>
</dependency>
3. Create a new Class called JsonReader and add a main method.
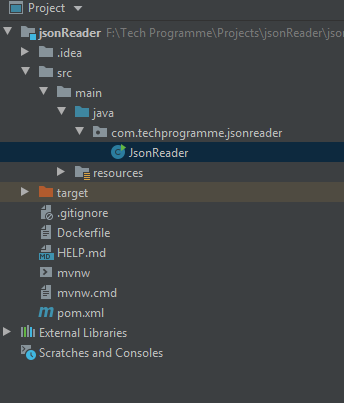
4. Change your JsonReader class as below.
Reader reader = Files.newBufferedReader(Paths.get(“E:\Projects\Music Lyrics\jSON\artist_json.json”)) will read the json file content. Map map = gson.fromJson(reader, Map.class) will map all the attributes & values as a map.
package com.techprogramme.jsonreader;
import com.google.gson.Gson;
import java.io.Reader;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Map;
public class JsonReader {
public static void main(String[] args) {
try {
Gson gson = new Gson();
Reader reader = Files.newBufferedReader(Paths.get("E:\\Projects\\Music Lyrics\\jSON\\artist_json.json"));
Map<?, ?> map = gson.fromJson(reader, Map.class);
String artistID = (String) map.get("artist_id");
System.out.println("Artist ID : "+artistID);
String artist_name = (String) map.get("artist_name");
System.out.println("Artist Name : "+artist_name);
String artist_category = (String) map.get("artist_category");
System.out.println("Artist Category : "+artist_category);
String artist_rating = (String) map.get("artist_rating");
System.out.println("Artist Rating : "+artist_rating);
ArrayList songList = (ArrayList) map.get("songs");
for (int i = 0; i < songList.size(); i++) {
Map<?, ?> s = (Map<?, ?>) songList.get(i);
String song_id = s.get("song_id").toString();
String song_name = s.get("song_name").toString();
System.out.println("Song ID : "+song_id+" || Song Name : "+song_name);
}
}catch (Exception ex){
ex.printStackTrace();
}
}
}
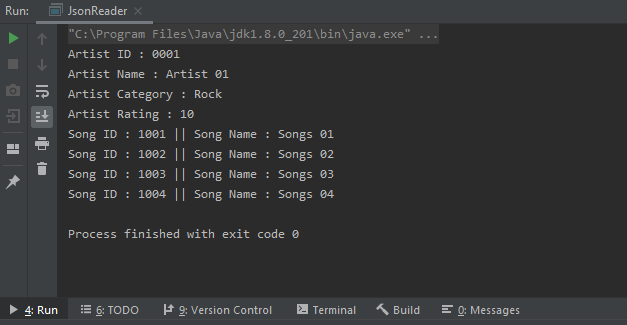
Download the source code from here