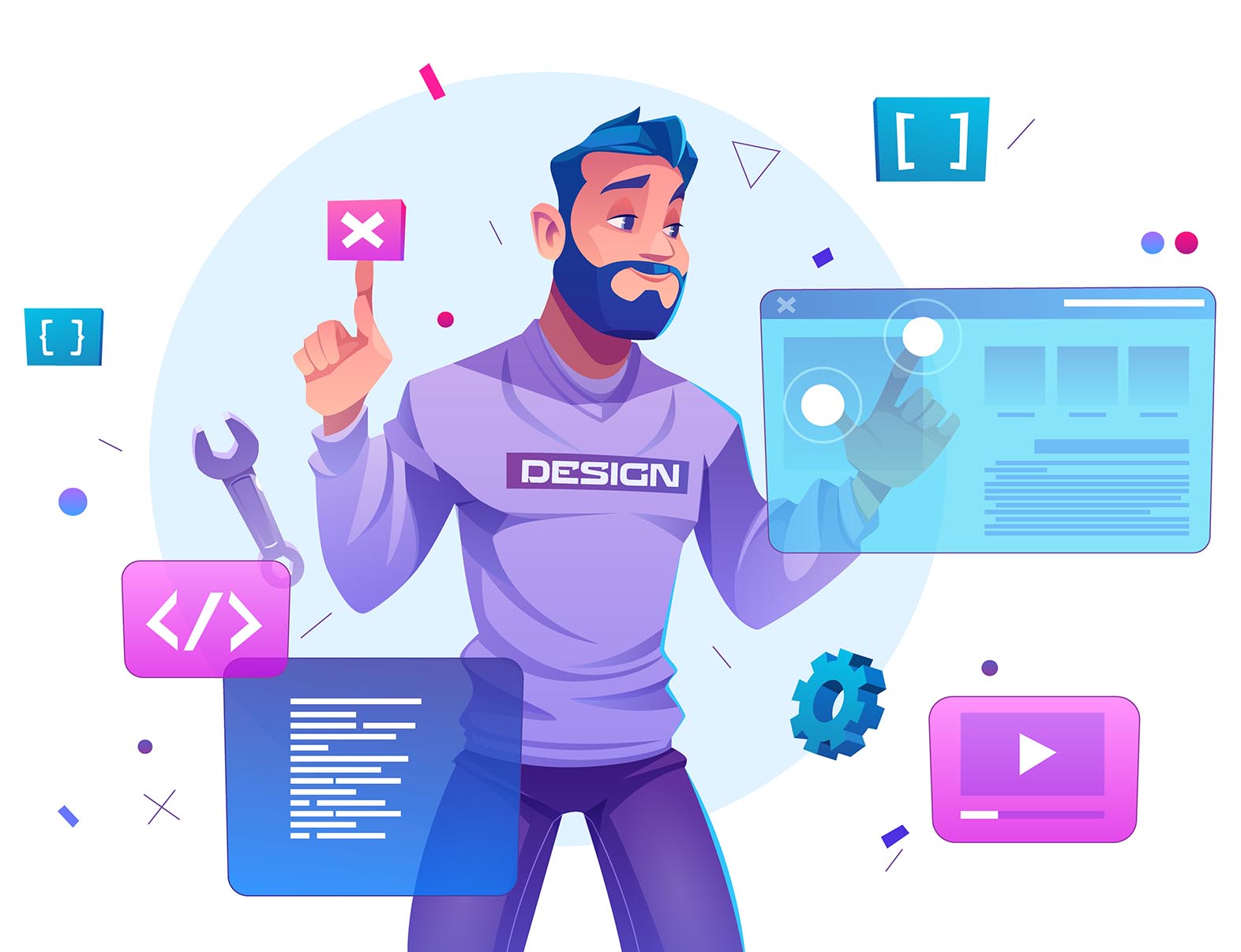
Java Socket Sever With Spring Boot | Intelij
Java Socket Programming can be use to create communication between applications which runs on different JVM’s. There are two type of Java Socket Programming connection types. Connection-Oriented and Connection-Less.
Here we are going to create a Socket Server and a Client to access the Socket Server with Spring Boot. In example we are going to pass a simple text from client to server. As per the example once the server is up, Socket Server also will be online and waiting for incoming requests
How to create a Socket Server
Lets create a new project first. Open Intelij and Click on File -> New -> Project or Click on New Project. Select Spring Initializr and click on Next.
Change the Group, Artifact, Name, Description and Package as you want. Once you complete click on Next
Click on Next from the dependency menu
Select the project location where you need to save your project
Create a New Class SocketServer and change it as below. onApplicationEvent will run the SocketServer programme once the spring boot server is up.
This code will initialize a new Socket Server and it will change to a state where the Socket Server is waiting for new requests
ServerSocket sock = new ServerSocket(port, 1);
m_sock = sock.accept();
Read the input stream of the data connection
InputStream is = m_sock.getInputStream();
InputStreamReader isReader = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isReader);
String data;
StringBuilder payload = new StringBuilder();
while (((data = br.readLine()) == null ? "" : data).length() != 0) {
payload.append(data);
}
SocketServer.java
package com.techprogramme.socketserver;
import org.springframework.boot.context.event.ApplicationReadyEvent;
import org.springframework.context.ApplicationListener;
import org.springframework.stereotype.Component;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Date;
@Component
public class SocketServer implements ApplicationListener<ApplicationReadyEvent> {
@Override
public void onApplicationEvent(final ApplicationReadyEvent event) {
startListener();
}
private void startListener() {
new Thread(() -> {
try {
int port = 8000;
ServerSocket sock = new ServerSocket(port, 1);
Socket m_sock;
while (true) {
try {
System.out.println("Socket Server listening on port: " + port);
m_sock = sock.accept();
System.out.println("New connection accepted " + m_sock.getInetAddress() + ":" + m_sock.getPort());
System.out.println("Accepted Time :" + new Date().toString());
InputStream is = m_sock.getInputStream();
InputStreamReader isReader = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isReader);
String data;
StringBuilder payload = new StringBuilder();
while (((data = br.readLine()) == null ? "" : data).length() != 0) {
payload.append(data);
}
System.out.println("Payload : " + payload.toString());
} catch (IOException e) {
System.out.println("failed to accept a connection, exception: " + e.getMessage());
continue;
} catch (Exception ex) {
System.out.println("Error: " + ex.getMessage());
continue;
}
m_sock.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}).start();
}
}
How to create a Socket Client
Create a New Class SocketClient and add a main function, since we need to run the client separately. Change the created Class as below
SocketClient.java
package com.techprogramme.socketserver;
import java.io.DataOutputStream;
import java.net.Socket;
public class SocketClient {
public static void main(String[] args) {
try {
String data = "Hello Socket Server";
Socket s = new Socket("localhost", 8000);
DataOutputStream dataOutputStream = new DataOutputStream(s.getOutputStream());
dataOutputStream.writeUTF(data);
dataOutputStream.flush();
dataOutputStream.close();
s.close();
System.out.println("\"" + data + "\"" + " Data sent to server");
} catch (Exception e) {
System.out.println(e);
}
}
}
Download the source code from here : https://github.com/TechProgramme/socket-server-spring-boot